IN THIS ARTICLE
PubNub is optimal for broadcasting updates to any number of concurrent subscribers. Using our APIs like Realtime Messaging and Presence, you can maintain state for a massive, interactive audience.
In this quick tutorial, we’ll use Presence, an API for online/offline detection of subscribers, to build an occupancy counter displaying how many users are online that updates in realtime as users join and leave. And we’ll do it in 15 lines of JavaScript.
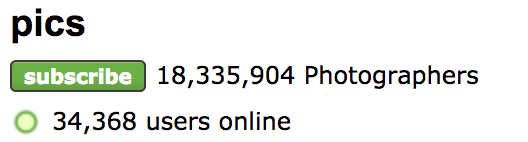
Reddit has a widget in their UI that show how many users are currently browsing a particular subreddit.
Realtime Occupancy Counter
You’ll first have to sign up for a PubNub account and create a project in the Admin Dashboard.
As we said, this widget utilizes the Presence API, which acts as an event emitter for the join, leave, timeout (and more) events of users that are connected to PubNub using your pub/sub keys. Enable the presence feature for your key and set the interval for the number of seconds between Presence updates. Make sure you click the save button at the bottom of the page once you have completed your configuration.
Next, let’s knock out the front-end to complete our fully-functioning widget. The current CSS forces the widget to sit fixed in the bottom left corner of the screen, even when the user scrolls down the page.
<!DOCTYPE html> <html> <head> <title>Currently Active Demo</title> <style type="text/css"> .currently-active { position: fixed; margin: 10px; padding: 5px; bottom: 0; left: 0; border: solid 1px #AFAFAF; border-radius: 6px; font-family: "Arial"; } </style> </head> <body> <div class="currently-active"> <span>Currently Active: </span><span id="active"></span> </div> </body> <script src="https://cdn.pubnub.com/sdk/javascript/pubnub.4.20.2.js"></script> <script type="text/javascript"> var active = document.getElementById('active'); function setCurrentlyActiveUsers(numberOfUsers) { active.innerText = numberOfUsers.toString(); } setCurrentlyActiveUsers(1); var pubnub = new PubNub({ publishKey : '__YOUR_PUB_KEY__', subscribeKey : '__YOUR_SUB_KEY__', heartbeatInterval: 30 }); pubnub.addListener({ presence: function(presenceEvent) { setCurrentlyActiveUsers(presenceEvent.occupancy); } }); pubnub.subscribe({ channels: ['myWebPage1'], withPresence: true }); </script> </html>
This is configurable to your needs. If your website is using a reactive framework like Angular, React, or Vue, I recommend using data binding to keep the number of users in sync with the data store.